Testing your code is very important.
Currently I'm having some python files which connects to sqlite database for user inputs and then performs some calculations which sets the output of the program. I'm new to python web programming and I want to know what is the best method to use python on web? Ex: I want to run my python files when the user clicks a button on the web page. I am having an problem in generating html report with HTMLTestRunner. I have created a test suite in a file called TestsSuite.py. Here's the code excerpt: import unittest from LoginTest import Log. This tool allows you to run any Python demo code online and helps you to test any python code from your browser without any configuration. This tool provides you any Python version from Python 2.7, Python 3.2, Python 3.3, Python 3.4, Python 3.5, Python 3.6, Python 3.7, Python 3.8 and runs your Python code in our sandbox environment. Online Python IDE is a web-based tool powered by ACE code editor. This tool can be used to learn, build, run, test your python script. You can open the script from your local and continue to build using this IDE. The test contains 40 questions and there is no time limit. The test is not official, it's just a nice way to see how much you know, or don't know, about HTML. Count Your Score. You will get 1 point for each correct answer. At the end of the Quiz, your total score will be displayed. Maximum score is 40 points.
Getting used to writing testing code and running this code in parallel is nowconsidered a good habit. Used wisely, this method helps to define yourcode’s intent more precisely and have a more decoupled architecture.
Some general rules of testing:
- A testing unit should focus on one tiny bit of functionality and prove itcorrect.
- Each test unit must be fully independent. Each test must be able to runalone, and also within the test suite, regardless of the order that they arecalled. The implication of this rule is that each test must be loaded witha fresh dataset and may have to do some cleanup afterwards. This isusually handled by
setUp()
andtearDown()
methods. - Try hard to make tests that run fast. If one single test needs more than afew milliseconds to run, development will be slowed down or the tests willnot be run as often as is desirable. In some cases, tests can’t be fastbecause they need a complex data structure to work on, and this data structuremust be loaded every time the test runs. Keep these heavier tests in aseparate test suite that is run by some scheduled task, and run all othertests as often as needed.
- Learn your tools and learn how to run a single test or a test case. Then,when developing a function inside a module, run this function’s testsfrequently, ideally automatically when you save the code.
- Always run the full test suite before a coding session, and run it againafter. This will give you more confidence that you did not break anythingin the rest of the code.
- It is a good idea to implement a hook that runs all tests before pushingcode to a shared repository.
- If you are in the middle of a development session and have to interruptyour work, it is a good idea to write a broken unit test about what youwant to develop next. When coming back to work, you will have a pointerto where you were and get back on track faster.
- The first step when you are debugging your code is to write a new testpinpointing the bug. While it is not always possible to do, those bugcatching tests are among the most valuable pieces of code in your project.
- Use long and descriptive names for testing functions. The style guide hereis slightly different than that of running code, where short names areoften preferred. The reason is testing functions are never called explicitly.
square()
or evensqr()
is ok in running code, but in testing code youwould have names such astest_square_of_number_2()
,test_square_negative_number()
. These function names are displayed whena test fails, and should be as descriptive as possible. - When something goes wrong or has to be changed, and if your code has agood set of tests, you or other maintainers will rely largely on thetesting suite to fix the problem or modify a given behavior. Thereforethe testing code will be read as much as or even more than the runningcode. A unit test whose purpose is unclear is not very helpful in thiscase.
- Another use of the testing code is as an introduction to new developers. Whensomeone will have to work on the code base, running and reading the relatedtesting code is often the best thing that they can do to start. They willor should discover the hot spots, where most difficulties arise, and thecorner cases. If they have to add some functionality, the first step shouldbe to add a test to ensure that the new functionality is not already aworking path that has not been plugged into the interface.
The Basics¶
unittest¶
unittest
is the batteries-included test module in the Python standardlibrary. Its API will be familiar to anyone who has used any of theJUnit/nUnit/CppUnit series of tools.
Creating test cases is accomplished by subclassing unittest.TestCase
.
As of Python 2.7 unittest also includes its own test discovery mechanisms.
Doctest¶
The doctest
module searches for pieces of text that look like interactivePython sessions in docstrings, and then executes those sessions to verify thatthey work exactly as shown.
Doctests have a different use case than proper unit tests: they are usuallyless detailed and don’t catch special cases or obscure regression bugs. Theyare useful as an expressive documentation of the main use cases of a module andits components. However, doctests should run automatically each time the fulltest suite runs.
A simple doctest in a function:
When running this module from the command line as in pythonmodule.py
, thedoctests will run and complain if anything is not behaving as described in thedocstrings.
Tools¶
py.test¶
py.test is a no-boilerplate alternative to Python’s standard unittest module.
Despite being a fully-featured and extensible test tool, it boasts a simplesyntax. Creating a test suite is as easy as writing a module with a couple offunctions:
and then running the py.test command:
is far less work than would be required for the equivalent functionality withthe unittest module!
Hypothesis¶
Hypothesis is a library which lets you write tests that are parameterized bya source of examples. It then generates simple and comprehensible examplesthat make your tests fail, letting you find more bugs with less work.
For example, testing lists of floats will try many examples, but report theminimal example of each bug (distinguished exception type and location):
Hypothesis is practical as well as very powerful and will often find bugsthat escaped all other forms of testing. It integrates well with py.test,and has a strong focus on usability in both simple and advanced scenarios.
tox¶
tox is a tool for automating test environment management and testing againstmultiple interpreter configurations.
tox allows you to configure complicated multi-parameter test matrices via asimple INI-style configuration file.
mock¶
unittest.mock
is a library for testing in Python. As of Python 3.3, it isavailable in thestandard library.
For older versions of Python:
It allows you to replace parts of your system under test with mock objects andmake assertions about how they have been used.
For example, you can monkey-patch a method:
To mock classes or objects in a module under test, use the patch
decorator.In the example below, an external search system is replaced with a mock thatalways returns the same result (but only for the duration of the test).
Mock has many other ways with which you can configure and control its behaviour.
Coverage.py is a tool for measuring code coverage of Python programs. Itmonitors your program, noting which parts of the code have been executed, thenanalyzes the source to identify code that could have been executed but was not.
Coverage measurement is typically used to gauge the effectiveness of tests. Itcan show which parts of your code are being exercised by tests, and which arenot.
The latest version is coverage.py 5.5, released February 28, 2021. It issupported on:
Python versions 2.7, 3.5, 3.6, 3.7, 3.8, 3.9, and 3.10 alpha.
PyPy2 7.3.3 and PyPy3 7.3.3.
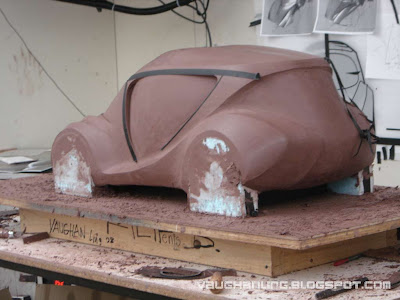
For Enterprise¶
Available as part of the Tidelift Subscription.
Coverage and thousands of other packages are working withTidelift to deliver one enterprise subscription that covers all of the opensource you use. If you want the flexibility of open source and the confidenceof commercial-grade software, this is for you. Learn more.
Quick start¶
Getting started is easy:
Install coverage.py:
For more details, see Installation.
Use
coveragerun
to run your test suite and gather data. However younormally run your test suite, you can run your test runner under coverage.If your test runner command starts with “python”, just replace the initial“python” with “coverage run”.Instructions for specific test runners:
If you usually use:
then you can run your tests under coverage with:
Many people choose to use the pytest-cov plugin, but for mostpurposes, it is unnecessary.
Change “python” to “coverage run”, so this:
becomes:
Nose has been unmaintained for a long time. You should seriouslyconsider adopting a different test runner.
Change this:
to:
To limit coverage measurement to code in the current directory, and alsofind files that weren’t executed at all, add the
--source=.
argument toyour coverage command line.Use
coveragereport
to report on the results:For a nicer presentation, use
coveragehtml
to get annotated HTMLlistings detailing missed lines:Then open htmlcov/index.html in your browser, to see areport like this.
Using coverage.py¶
There are a few different ways to use coverage.py. The simplest is thecommand line, which lets you run your program and see the results.If you need more control over how your project is measured, you can use theAPI.
Some test runners provide coverage integration to make it easy to usecoverage.py while running tests. For example, pytest has the pytest-covplugin.
You can fine-tune coverage.py’s view of your code by directing it to ignoreparts that you know aren’t interesting. See Specifying source files and Excluding code from coverage.pyfor details.
Getting help¶
If the FAQ doesn’t answer your question, you can discusscoverage.py or get help using it on the Testing In Python mailing list.
Bug reports are gladly accepted at the GitHub issue tracker.GitHub also hosts the code repository.
Professional support for coverage.py is available as part of the TideliftSubscription.
I can be reached in a number of ways. I’m happy to answer questions aboutusing coverage.py.